This allows you to replace default checkboxes with a nicer-looking custom font alternative. This example uses Font Awesome 4 icons, but the CSS could easily be modified to use any font. For the code below to work as-is, Font Awesome 4 must already be available on the page.
This example uses two types of icons:
class: fancy-checkbox
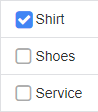
class: fancy-checkbox-toggle
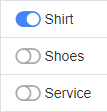
HTML & CSS are included below for native checkboxes, and further down there is a separate section containing the HTML & CSS for ASP.Net WebForms CheckBox and CheckBoxList controls.
Native HTML Checkbox
Fancy Checkbox:
<label class="fancy-checkbox">
<input type="checkbox" id="chkTest" value="1" name="chkTest" />
<label for="chkTest">Check Box</label>
</label>
Fancy Toggle Switch:
<label class="fancy-checkbox-toggle">
<input type="checkbox" id="chkTest" value="1" name="chkTest" />
<label for="chkTest">Toggle Switch</label>
</label>
Fancy Checkbox CSS:
.fancy-checkbox {
position: relative;
display: block;
padding: 0 0 0 20px;
margin: 0;
cursor: pointer;
/* Make text & control un-selectable, otherwise it will
sometimes get selected if the user repeatedly clicks quickly */
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.fancy-checkbox input {
display: none;
}
.fancy-checkbox label {
cursor: pointer;
padding-left: 0;
}
.fancy-checkbox input[type="checkbox"] + label:before {
position: absolute;
top: 1px;
left: 0;
font-family: FontAwesome;
content: "\f096";
font-size: 20px;
line-height: 1;
color: #aaa;
}
.fancy-checkbox input[type="checkbox"]:checked + label:before {
content: "\f14a";
color: #4285f4;
}
Fancy Toggle Switch CSS:
.fancy-checkbox-toggle {
position: relative;
display: block;
padding: 0 0 0 29px;
margin: 0;
cursor: pointer;
/* Make text & control un-selectable, otherwise it will
sometimes get selected if the user repeatedly clicks quickly */
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.fancy-checkbox-toggle input {
display: none;
}
.fancy-checkbox-toggle label {
cursor: pointer;
padding-left: 0;
}
.fancy-checkbox-toggle input[type="checkbox"] + label:before {
position: absolute;
top: -1px;
left: 0;
font-family: FontAwesome;
content: "\f204";
font-size: 22px;
line-height: 1;
color: #aaa;
}
.fancy-checkbox-toggle input[type="checkbox"]:checked + label:before {
content: "\f205";
color: #4285f4;
}
ASP.Net CheckBox & CheckBoxList Controls
HTML for Fancy Checkbox (renders in a span):
<asp:CheckBox ID="chkSelect" runat="server" Text="Check Box" CssClass="fancy-checkbox"/>
HTML for Fancy Checkboxes in a CheckBoxList (renders in a table):
<asp:CheckBoxList ID="CheckBoxList1" runat="server" CssClass="fancy-checkbox">
<asp:ListItem Selected="true">People</asp:ListItem>
<asp:ListItem>Animals</asp:ListItem>
<asp:ListItem Enabled="false">Scientists</asp:ListItem>
</asp:CheckBoxList>
HTML for Fancy Toggle Switch (renders in a span):
<asp:CheckBox ID="chkSelect" runat="server" Text="Toggle Switch" CssClass="fancy-checkbox-toggle"/>
HTML for Fancy Toggle Switches in a CheckBoxList (renders in a table):
<asp:CheckBoxList ID="CheckBoxList1" runat="server" CssClass="fancy-checkbox-toggle">
<asp:ListItem Selected="true">People</asp:ListItem>
<asp:ListItem>Animals</asp:ListItem>
<asp:ListItem Enabled="false">Scientists</asp:ListItem>
</asp:CheckBoxList>
Note: The Text
property must be populated (Text=" "
at minimum) for the ASP.Net CheckBox control so that the <label>
tag is rendered inside the control. The fancy checkbox is rendered before the label using CSS :before
, so the label must be present. If you do not populate the Text
property, there will be nothing to replace the hidden native checkbox.
CSS for ASP.Net Fancy Checkbox (in CheckBox and CheckBoxList Controls):
/* ASP.Net CheckBox Control - Rendered in a SPAN */
span.fancy-checkbox {
position: relative;
display: block;
padding: 0 0 0 20px;
cursor: pointer;
/* Make text & control un-selectable, otherwise it will
sometimes get selected if the user repeatedly clicks quickly */
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
/* ASP.Net CheckBoxList - Rendered in a TABLE */
table.fancy-checkbox td {
position: relative;
padding-left: 20px;
/* Make text & control un-selectable, otherwise it will
sometimes get selected if the user repeatedly clicks quickly */
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.fancy-checkbox input {
display: none;
}
.fancy-checkbox label {
cursor: pointer;
padding-left: 0;
}
.fancy-checkbox input[type="checkbox"] + label:before {
position: absolute;
top: 1px;
left: 0;
font-family: FontAwesome;
content: "\f096";
font-size: 20px;
line-height: 1;
color: #aaa;
}
.fancy-checkbox input[type="checkbox"]:checked + label:before {
content: "\f14a";
color: #4285f4;
font-size: 18px; /* Shrink checked icon slightly to match unchecked */
}
.fancy-checkbox input[type="checkbox"]:disabled + label:before {
color: #ccccdd;
}
.fancy-checkbox.aspNetDisabled label,
.fancy-checkbox .aspNetDisabled label {
cursor: not-allowed;
}
CSS for ASP.Net Fancy Toggle Switch (in CheckBox and CheckBoxList Controls):
span.fancy-checkbox-toggle {
position: relative;
display: block;
padding: 0 0 0 29px;
cursor: pointer;
/* Make text & control un-selectable, otherwise it will
sometimes get selected if the user repeatedly clicks quickly */
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
/* ASP.Net CheckBoxList - Rendered in a TABLE */
table.fancy-checkbox-toggle td {
position: relative;
padding-left: 29px;
/* Make text & control un-selectable, otherwise it will
sometimes get selected if the user repeatedly clicks quickly */
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.fancy-checkbox-toggle input {
display: none;
}
.fancy-checkbox-toggle label {
cursor: pointer;
padding-left: 0;
font-weight: normal;
}
.fancy-checkbox-toggle input[type="checkbox"] + label:before {
position: absolute;
top: -1px;
left: 0;
font-family: FontAwesome;
content: "\f204";
font-size: 22px;
line-height: 1;
color: #aaa;
}
.fancy-checkbox-toggle input[type="checkbox"]:checked + label:before {
content: "\f205";
color: #4285f4;
}
.fancy-checkbox-toggle input[type="checkbox"]:disabled + label:before {
color: #ccccdd;
}
.fancy-checkbox-toggle.aspNetDisabled label,
.fancy-checkbox-toggle .aspNetDisabled label {
cursor: not-allowed;
}
Based on the following article: http://thatwebdude.com/tutorial/bigger-html-checkboxes-with-font-awesome-and-css/